Predictive back gesture on Android 14 – Everything you need to know
Table of Contents
Upgrade your Android app to support future predictive back gesture
In this article, we will see how to enable predictive back gesture on Android 14 and above. But before jumping to the implementation part we will first see what predictive back gesture is.
Predictive back gesture lets users preview the result of a Back gesture before they fully complete it. Though the support was added in Android 13, this feature was not visible to the users, by default. It can only be enabled from developer options for testing. Check the animation below to get a better understanding of the behavior of predictive back gesture:
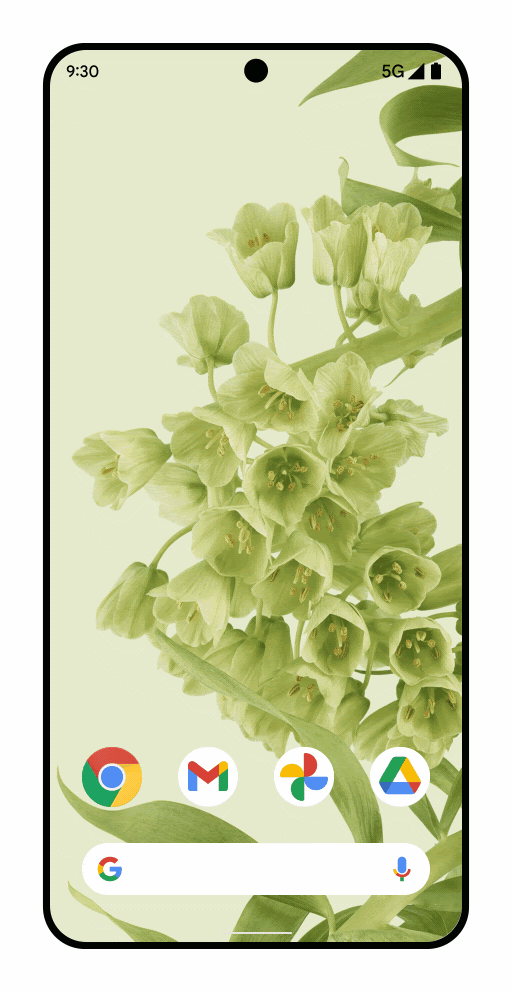
Change in the behavior of back navigation is done based on users’ feedback as mentioned in the android-developers blog:
Since we introduced gesture navigation in Android 10, users have signaled they want to understand where a back gesture will take them before they complete it.
https://android-developers.googleblog.com/2022/07/prepare-your-app-to-support-predictive-back-gestures.html
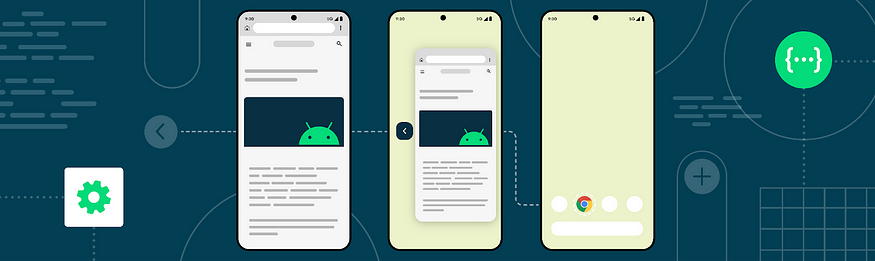
Enabling the Predictive Back gesture for an app
In Android 13, it is optional for app developers to add support for predictive back gesture for their app but in future releases, this may change.
Note: Android 13 doesn’t make the predictive back gesture visible to users, but it does provide an early version of the UI as a developer option for testing. We plan to make this UI available to users in a future Android release. In the meantime, we strongly recommend updating your app to ensure you get the latest updates.
https://developer.android.com/guide/navigation/custom-back/predictive-back-gesture
So Let us go through the set of changes that are required to enable it:
- Update SDK Versions in build.gradle
Update thetargetSdk
andcompileSdk
versions to 33 in build.gradle file - Add support in the Manifest file
In AndroidManifest.xml, enable the support for the predictive back gesture for your app by adding the following attribute in the application tag:android:enableOnBackInvokedCallback=”true”
- Add/upgrade the Androidx Activity dependency
Need to add Androidx Activity dependency in build.gradle. This change is required to make sure that the other components like Fragments, Navigation Components, etc. work seamlessly with the back navigation gesture.
Following dependency needs to be added in build.gradle:implementation “androidx.activity:activity:1.6.0-alpha05”
- Replace deprecated onBackPressed() with OnBackPressedCallback implementation
Since we have enabled the support for Predictive Back Gesture for the app,onBackPressed()
method will no longer get the system callback when a user performs a back gesture. So we need to move the code fromonBackPressed()
method tohandleOnBackPressed()
method ofOnBackPressedCallback
class. Code changes are documented in another section below - Testing it on Android Device
On Android 13, the predictive back gesture is disabled by default. So to test the app, we need to enable it from developer options.
On an Android device, go to Settings > System > Developer options. Then search for Predictive back animations and enable it
We are done with the changes and are good to test the app on an Android device.
To support predictive back gestures, some Android native APIs have also been modified. As a part of these changes, onBackPressed()
method has been deprecated and needs to be replaced with the other alternative APIs. In this article, we will see how to replace deprecated onBackPressed()
implementation with OnBackPressedCallback
based implementation. onBackPressed()
has been deprecated in the Android 13 release and the reason to deprecate this is to enable the support for the future predictive back gesture.
Replacing deprecated onBackPressed() with OnBackPressedCallback implementation
To replace onBackPressed()
we can either use OnBackPressedCallback
or OnBackInvokedCallback
. It is recommended to use OnBackPressedCallback
, as it is a part of Androidx so the implementation will be backward compatible. OnBackInvokedCallback
is a part of Platform API and Added in API 33 so this will not work if we want to support devices below API Level 33.
Refer code snippets below to see the implementation in both ways:
activity?.onBackPressedDispatcher?.addCallback(viewLifecycleOwner, object: OnBackPressedCallback(true){ override fun handleOnBackPressed() { //Add back handling code here: } })
activity?.onBackInvokedDispatcher?.registerOnBackInvokedCallback(OnBackInvokedDispatcher.PRIORITY_DEFAULT){ Log.d("MainActivity", "Back Pressed callback in OnBackInvokedCallback") }
An important thing to notice here is that the callbacks are always executed in reverse order. Meaning the fragment which registered the onBackPressedCallback
last will get the first callback when a user swipes back
I hope this tutorial helps. If it did, do leave a comment below
References:
https://developer.android.com/guide/navigation/custom-back/predictive-back-gesture
https://android-developers.googleblog.com/2022/07/prepare-your-app-to-support-predictive-back-gestures.html